Using the SDK
Overview
- SDK offers a programmatic way of interacting with the Stacktape API.
- Unlike other Infastructure as Code tools such as AWS CDK or Pulumi, it offers a truly dynamic way of interacting with your stacks (as opposed to just programmatically creating the configuration).
- Behind the scenes, Stacktape SDK interacts with a GRPC server that executes Stacktape commands in a separate process.
- Currently, the SDK is available only for Javascript/Typescript. Python, Go and Java SDKs will be available soon.
- Stacktape SDK is fully typed and includes a built-in documentation for every property.
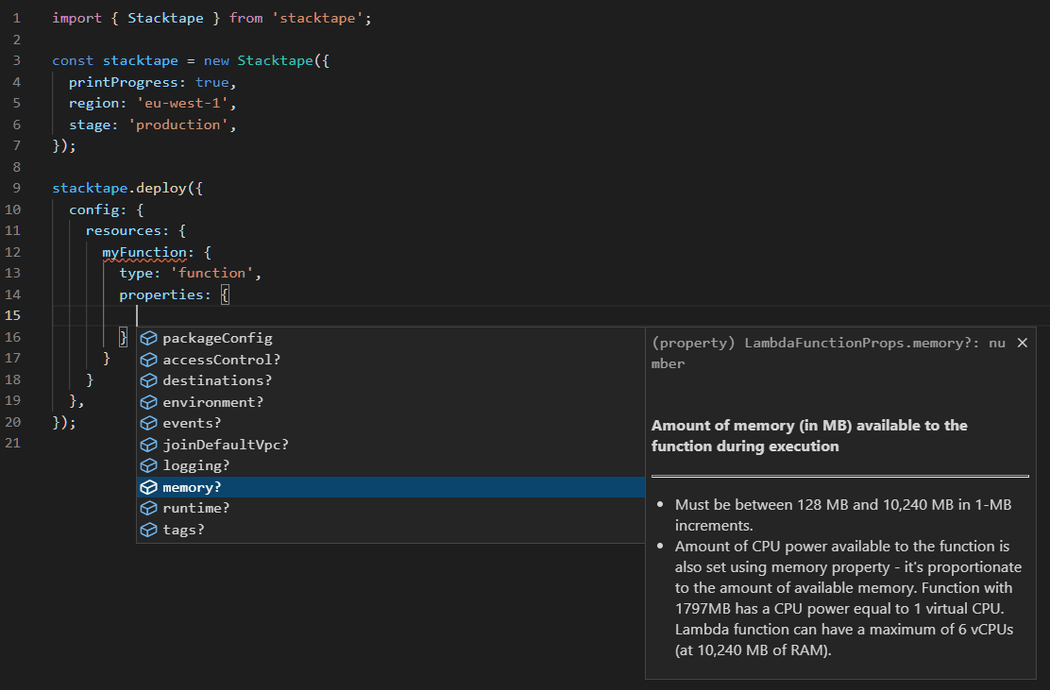
Example usage
Copy
import { Stacktape } from 'stacktape';const stacktape = new Stacktape({region: 'eu-west-1',stage: 'production'});const run = async () => {await Promise.all([stacktape.deploy({config: {serviceName: 'stack-1',resources: [/* ...your resources... */]}}),stacktape.deploy({config: {serviceName: 'stack-2',resources: [/* ...your resources... */]}})]);};run();
Example script written in typescript that concurrently deploys 2 different stacks.
Print progress
- To print information about the operations that Stacktape performs, you can set the
printProgress
property.
Copy
const stacktape = new Stacktape({printProgress: true});
Subscribing to events
- Stacktape process sends events that describe what's currently happening. You can subscribe to these event
Copy
const stacktape = new Stacktape({onEvent: (eventData) => {// do something with the event data}});